
3 Ways to Square a Number in JavaScript
Squaring a number in JavaScript is a fundamental operation that involves multiplying the number by itself. So, how to square a number in JavaScript?
This can be achieved through various methods, such as using the arithmetic operator *
, the Math.pow()
function, or the exponentiation operator **
. Each method has its use case depending on the context and readability preferences.
How do you use the multiplication operator to square a number in JavaScript?
The most straightforward way to square a number is by using the multiplication operator (*
). This method is clear and concise, making it easy to understand for anyone familiar with basic arithmetic operations.
const number = 5; const squared = number * number; console.log(squared); // Outputs: 25
What is Math.pow()
and how to use it?
You can useMath.pow()
for a more explicit approach. This function takes two arguments: the base and the exponent. To square a number, you would set the exponent to 2. This method is particularly useful when dealing with variable exponents or when the code needs to be explicitly clear about the operation being performed.
const number = 5; const squared = Math.pow(number, 2); console.log(squared); // Outputs: 25
How does the exponentiation operator work in JS?
JavaScript ES6 introduced the exponentiation operator (**
), providing a more concise syntax for performing exponentiation. This operator is particularly useful for readability and writing concise code, especially when dealing with complex mathematical operations.
const number = 5; const squared = number ** 2; console.log(squared); // Outputs: 25
Each of these methods can be used to square a number in JavaScript, with the choice depending on your specific needs and coding style preferences. Whether you prefer the clarity of Math.pow()
, the simplicity of the multiplication operator, or the modern syntax of the exponentiation operator, JavaScript provides flexible options to suit different scenarios.
Invite only
We're building the next generation of data visualization.
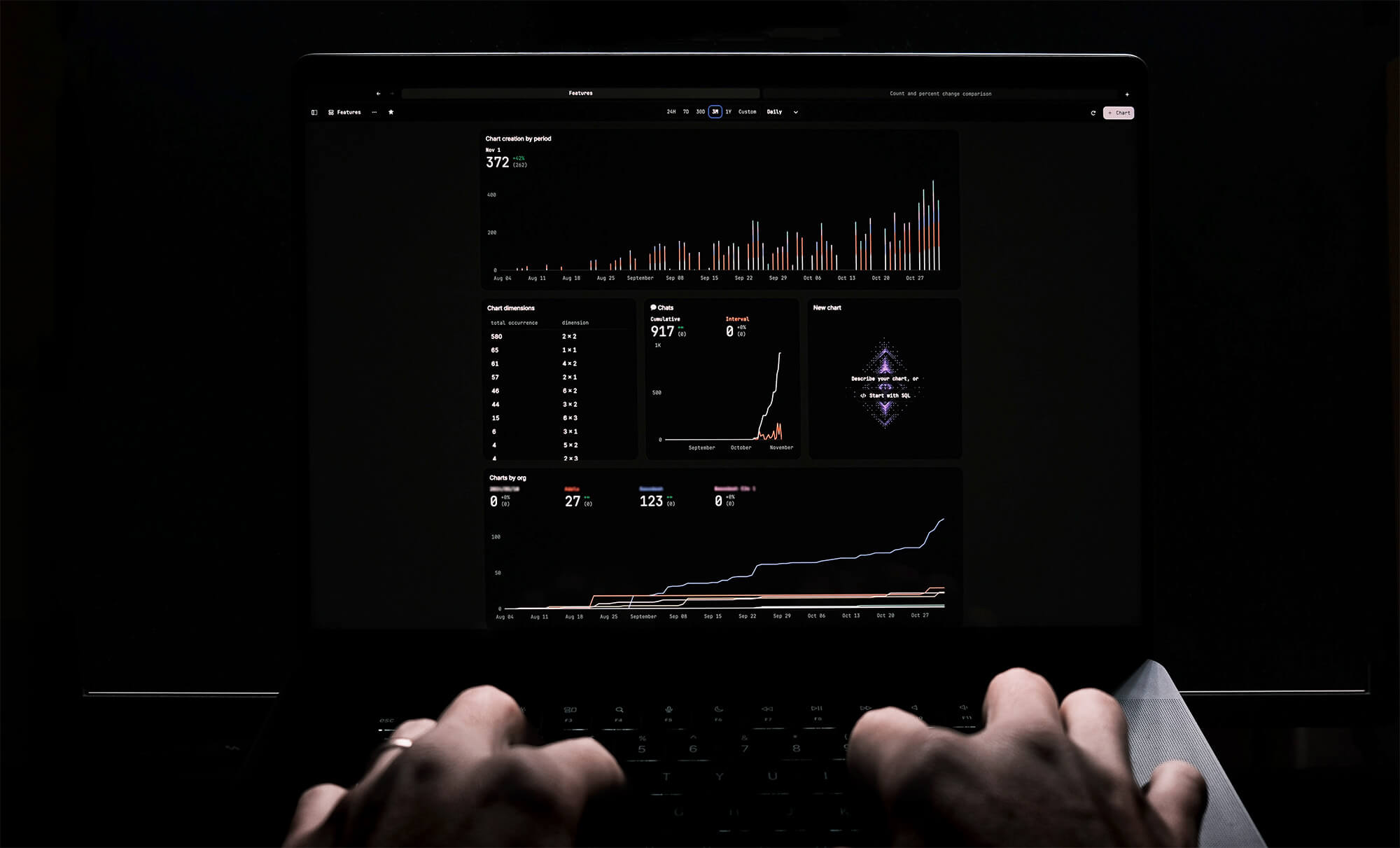
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper