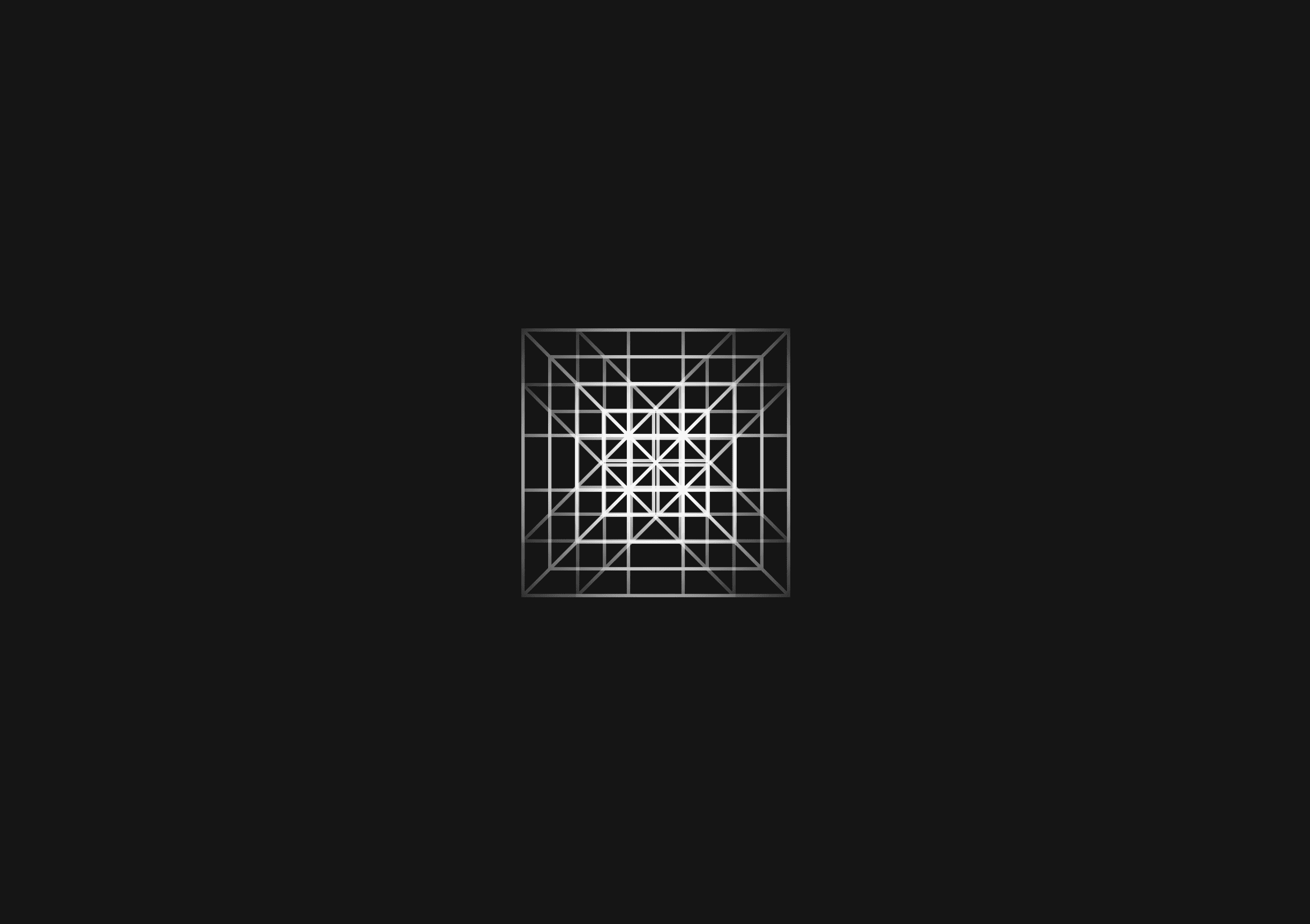
How to Check if a Number is a Float in JavaScript
November 8, 2023
In order to determine if a number if a float in JavaScript, you need to understandthe nature of number representation in the language. Since JavaScript uses IEEE 754 standard for floating-point arithmetic, integers and floats are not distinct types. But there are techniques to differentiate them. We’ll cover them below.
Understanding number representation in JavaScript
In JavaScript, numbers are represented as double-precision 64-bit binary format IEEE 754 values, which means that both integers and floating-point numbers are treated as the same type. This can make it challenging to determine if a number is an integer or a float, as they are not inherently distinguished.
Using the Number
object and parseInt
The Number
object provides methods that can be used to inspect the characteristics of numeric values. One approach to check if a number is a float is to compare it with its integer part obtained using parseInt
.
function isFloat(n) { return Number(n) === n && n % 1 !== 0; }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Leveraging parseFloat
and strict comparison
Alternatively, parseFloat
can be used to ensure the number remains the same when its floating-point representation is parsed from a string. Strict comparison to the original number then reveals its type.
function isFloat(n) { return parseFloat(n) === n && !Number.isInteger(n); }
Utilizing Math.floor
or Math.ceil
Mathematical functions like Math.floor
or Math.ceil
can also be used to check for float numbers by comparing the original number with the result of the floor or ceil operation.
function isFloat(n) { return Math.floor(n) !== n; }
Employing Number.isInteger
A straightforward method provided by ES6 is Number.isInteger
, which directly checks if a number is an integer, hence its negation would imply a float.
function isFloat(n) { return !Number.isInteger(n); }
TOC
November 8, 2023
In order to determine if a number if a float in JavaScript, you need to understandthe nature of number representation in the language. Since JavaScript uses IEEE 754 standard for floating-point arithmetic, integers and floats are not distinct types. But there are techniques to differentiate them. We’ll cover them below.
Understanding number representation in JavaScript
In JavaScript, numbers are represented as double-precision 64-bit binary format IEEE 754 values, which means that both integers and floating-point numbers are treated as the same type. This can make it challenging to determine if a number is an integer or a float, as they are not inherently distinguished.
Using the Number
object and parseInt
The Number
object provides methods that can be used to inspect the characteristics of numeric values. One approach to check if a number is a float is to compare it with its integer part obtained using parseInt
.
function isFloat(n) { return Number(n) === n && n % 1 !== 0; }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Leveraging parseFloat
and strict comparison
Alternatively, parseFloat
can be used to ensure the number remains the same when its floating-point representation is parsed from a string. Strict comparison to the original number then reveals its type.
function isFloat(n) { return parseFloat(n) === n && !Number.isInteger(n); }
Utilizing Math.floor
or Math.ceil
Mathematical functions like Math.floor
or Math.ceil
can also be used to check for float numbers by comparing the original number with the result of the floor or ceil operation.
function isFloat(n) { return Math.floor(n) !== n; }
Employing Number.isInteger
A straightforward method provided by ES6 is Number.isInteger
, which directly checks if a number is an integer, hence its negation would imply a float.
function isFloat(n) { return !Number.isInteger(n); }
November 8, 2023
In order to determine if a number if a float in JavaScript, you need to understandthe nature of number representation in the language. Since JavaScript uses IEEE 754 standard for floating-point arithmetic, integers and floats are not distinct types. But there are techniques to differentiate them. We’ll cover them below.
Understanding number representation in JavaScript
In JavaScript, numbers are represented as double-precision 64-bit binary format IEEE 754 values, which means that both integers and floating-point numbers are treated as the same type. This can make it challenging to determine if a number is an integer or a float, as they are not inherently distinguished.
Using the Number
object and parseInt
The Number
object provides methods that can be used to inspect the characteristics of numeric values. One approach to check if a number is a float is to compare it with its integer part obtained using parseInt
.
function isFloat(n) { return Number(n) === n && n % 1 !== 0; }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Leveraging parseFloat
and strict comparison
Alternatively, parseFloat
can be used to ensure the number remains the same when its floating-point representation is parsed from a string. Strict comparison to the original number then reveals its type.
function isFloat(n) { return parseFloat(n) === n && !Number.isInteger(n); }
Utilizing Math.floor
or Math.ceil
Mathematical functions like Math.floor
or Math.ceil
can also be used to check for float numbers by comparing the original number with the result of the floor or ceil operation.
function isFloat(n) { return Math.floor(n) !== n; }
Employing Number.isInteger
A straightforward method provided by ES6 is Number.isInteger
, which directly checks if a number is an integer, hence its negation would imply a float.
function isFloat(n) { return !Number.isInteger(n); }
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Remove Characters from a String in JavaScript
Jeremy Sarchet



How to Sort Strings in JavaScript
Max Musing



How to Remove Spaces from a String in JavaScript
Jeremy Sarchet



Detecting Prime Numbers in JavaScript
Robert Cooper



How to Parse Boolean Values in JavaScript
Max Musing



How to Remove a Substring from a String in JavaScript
Robert Cooper