
How to Check if a Table Exists in MySQL
November 13, 2023
Checking if a table exists in MySQL is essential for database management and script writing, ensuring that operations like table creation or data insertion are performed only if the table does not already exist. This guide provides clear methods for verifying the existence of a table in MySQL databases, tailored for engineers seeking efficient and reliable solutions.
Understand the information_schema database
MySQL maintains a special database called information_schema
that contains metadata about all other databases and tables. To check if a particular table exists, you can query this database.
Query the information_schema.tables
Use the EXISTS
clause in conjunction with a SELECT
statement to check for a table's existence. Replace your_table_name
with the name of your table and your_database_name
with the name of your database.
SELECT EXISTS ( SELECT * FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' );
Interpret the query result
The result of the above query is binary:
1
indicates the table exists.0
indicates it does not.
Use SHOW TABLES command
Another method is to use the SHOW TABLES
command, which lists all tables in a specific database.
Execute the SHOW TABLES command
Run the following command in MySQL, where your_database_name
is your database's name.
SHOW TABLES FROM your_database_name LIKE 'your_table_name';
Analyze the output
- If the table exists, this command returns its name.
- If the table doesn't exist, the result is an empty set.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Utilize conditional statements in scripts
In scripting scenarios, use conditional logic to check for table existence before performing operations.
Example of conditional creation
This SQL script checks for a table's existence before creating it.
SET @tbl_exists = ( SELECT COUNT(*) FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' ); SET @sql = IF(@tbl_exists = 0, 'CREATE TABLE your_database_name.your_table_name (...)', 'SELECT "Table already exists"' ); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt;
This script uses MySQL's prepared statement functionality to conditionally create a table only if it does not already exist.
Conclusion
Employing these methods ensures robust database operations, avoiding errors related to existing tables and maintaining optimal script performance. Whether querying the information_schema
database, using the SHOW TABLES
command, or incorporating conditional logic in scripts, these approaches are foundational for effective MySQL database management.
TOC
November 13, 2023
Checking if a table exists in MySQL is essential for database management and script writing, ensuring that operations like table creation or data insertion are performed only if the table does not already exist. This guide provides clear methods for verifying the existence of a table in MySQL databases, tailored for engineers seeking efficient and reliable solutions.
Understand the information_schema database
MySQL maintains a special database called information_schema
that contains metadata about all other databases and tables. To check if a particular table exists, you can query this database.
Query the information_schema.tables
Use the EXISTS
clause in conjunction with a SELECT
statement to check for a table's existence. Replace your_table_name
with the name of your table and your_database_name
with the name of your database.
SELECT EXISTS ( SELECT * FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' );
Interpret the query result
The result of the above query is binary:
1
indicates the table exists.0
indicates it does not.
Use SHOW TABLES command
Another method is to use the SHOW TABLES
command, which lists all tables in a specific database.
Execute the SHOW TABLES command
Run the following command in MySQL, where your_database_name
is your database's name.
SHOW TABLES FROM your_database_name LIKE 'your_table_name';
Analyze the output
- If the table exists, this command returns its name.
- If the table doesn't exist, the result is an empty set.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Utilize conditional statements in scripts
In scripting scenarios, use conditional logic to check for table existence before performing operations.
Example of conditional creation
This SQL script checks for a table's existence before creating it.
SET @tbl_exists = ( SELECT COUNT(*) FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' ); SET @sql = IF(@tbl_exists = 0, 'CREATE TABLE your_database_name.your_table_name (...)', 'SELECT "Table already exists"' ); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt;
This script uses MySQL's prepared statement functionality to conditionally create a table only if it does not already exist.
Conclusion
Employing these methods ensures robust database operations, avoiding errors related to existing tables and maintaining optimal script performance. Whether querying the information_schema
database, using the SHOW TABLES
command, or incorporating conditional logic in scripts, these approaches are foundational for effective MySQL database management.
November 13, 2023
Checking if a table exists in MySQL is essential for database management and script writing, ensuring that operations like table creation or data insertion are performed only if the table does not already exist. This guide provides clear methods for verifying the existence of a table in MySQL databases, tailored for engineers seeking efficient and reliable solutions.
Understand the information_schema database
MySQL maintains a special database called information_schema
that contains metadata about all other databases and tables. To check if a particular table exists, you can query this database.
Query the information_schema.tables
Use the EXISTS
clause in conjunction with a SELECT
statement to check for a table's existence. Replace your_table_name
with the name of your table and your_database_name
with the name of your database.
SELECT EXISTS ( SELECT * FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' );
Interpret the query result
The result of the above query is binary:
1
indicates the table exists.0
indicates it does not.
Use SHOW TABLES command
Another method is to use the SHOW TABLES
command, which lists all tables in a specific database.
Execute the SHOW TABLES command
Run the following command in MySQL, where your_database_name
is your database's name.
SHOW TABLES FROM your_database_name LIKE 'your_table_name';
Analyze the output
- If the table exists, this command returns its name.
- If the table doesn't exist, the result is an empty set.
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Utilize conditional statements in scripts
In scripting scenarios, use conditional logic to check for table existence before performing operations.
Example of conditional creation
This SQL script checks for a table's existence before creating it.
SET @tbl_exists = ( SELECT COUNT(*) FROM information_schema.tables WHERE table_schema = 'your_database_name' AND table_name = 'your_table_name' ); SET @sql = IF(@tbl_exists = 0, 'CREATE TABLE your_database_name.your_table_name (...)', 'SELECT "Table already exists"' ); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt;
This script uses MySQL's prepared statement functionality to conditionally create a table only if it does not already exist.
Conclusion
Employing these methods ensures robust database operations, avoiding errors related to existing tables and maintaining optimal script performance. Whether querying the information_schema
database, using the SHOW TABLES
command, or incorporating conditional logic in scripts, these approaches are foundational for effective MySQL database management.
What is Basedash?
What is Basedash?
What is Basedash?
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
Basedash is the best MySQL admin panel
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.
If you're building with MySQL, you need Basedash. It gives you an instantly generated admin panel to understand, query, build dashboards, edit, and share access to your data.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Add Columns to MySQL Tables with ALTER TABLE
Robert Cooper



How to Add Columns to Your MySQL Table
Max Musing



Pivot Tables in MySQL
Robert Cooper



How to Rename a Table in MySQL
Max Musing
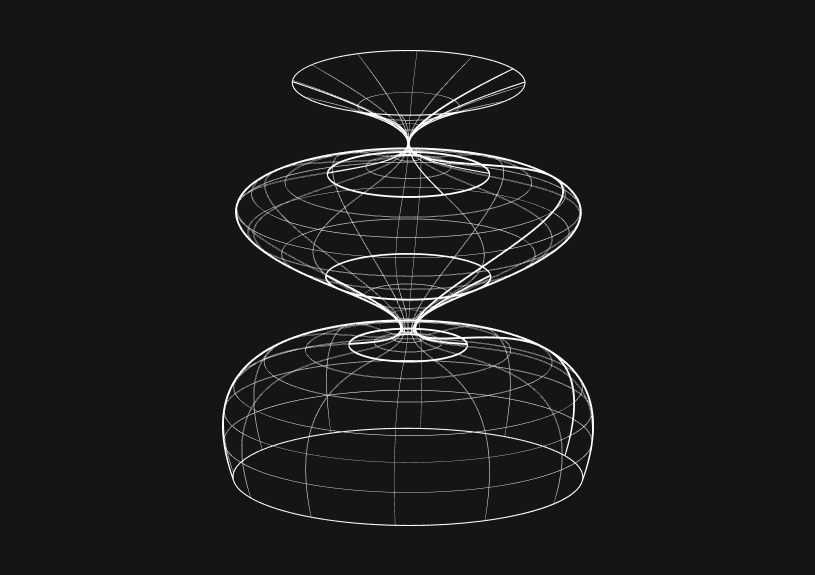
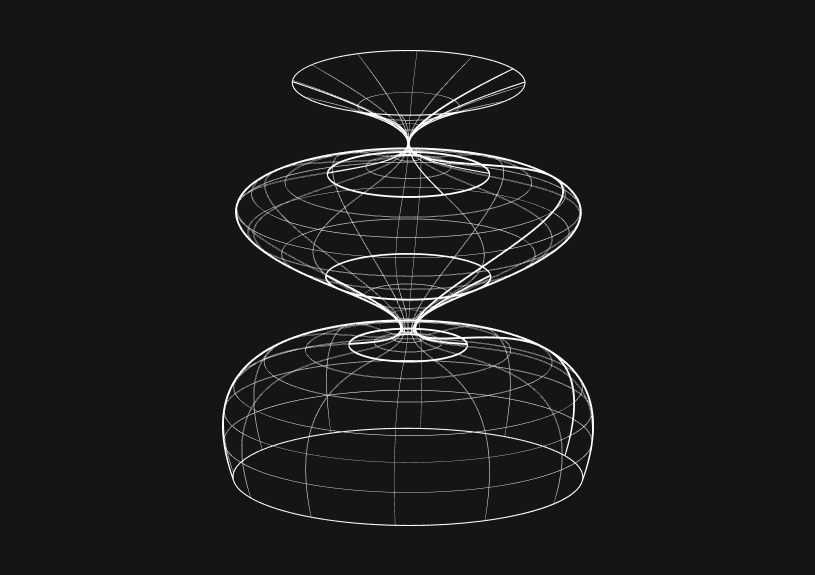
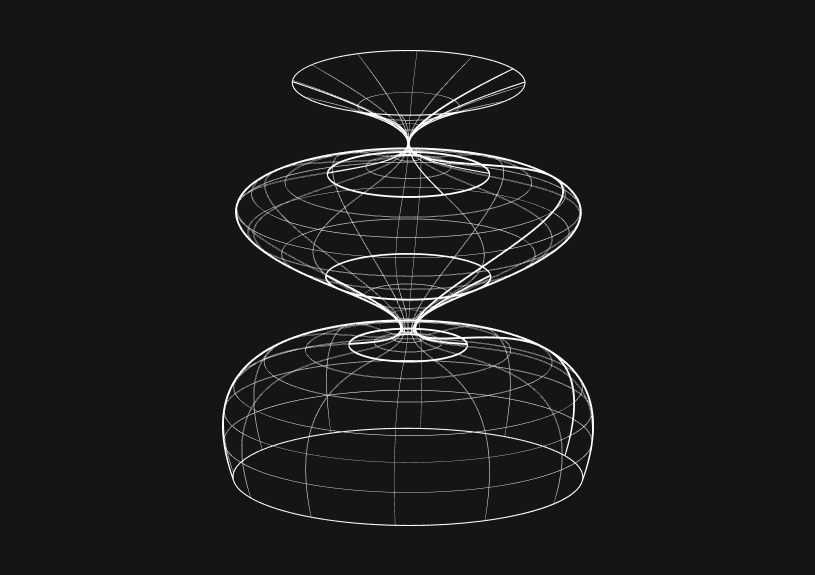
How to Optimize MySQL Tables for Better Performance
Robert Cooper



How to Display MySQL Table Schema: A Guide
Jeremy Sarchet