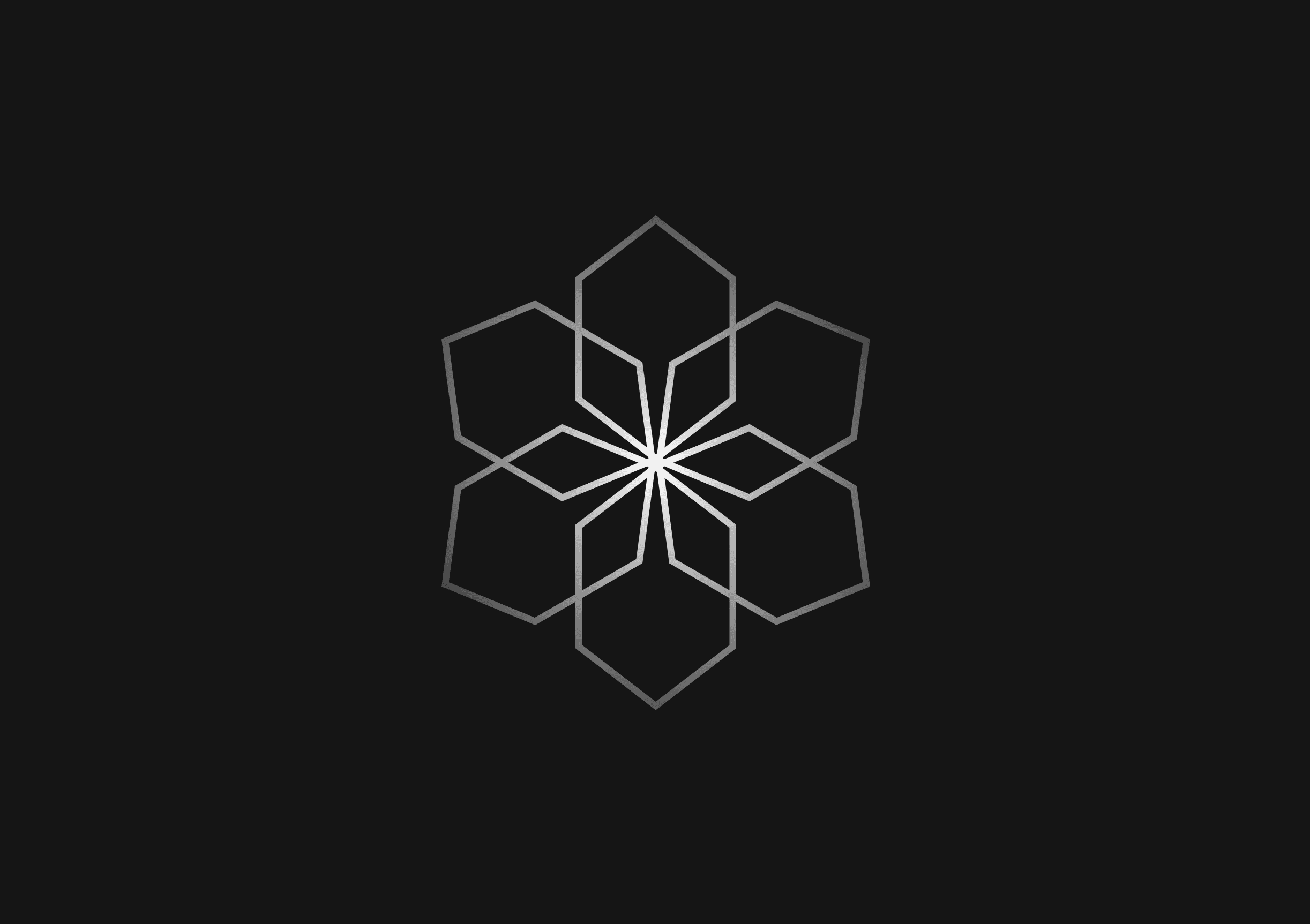
How to fix the "split is not a function" error in JavaScript
November 7, 2023
"split is not a function" is an error thrown in JavaScript when the split
method is called on a value that is not a string. If you keep getting “split is not a function,” keep reading. This guide walks you through why this error occurs and how to resolve it.
Understanding the split function
The split()
method in JavaScript is used to divide a string into an ordered list of substrates, puts these substrates into an array, and returns the array. It's important to note that split()
is a string method:
const str = "hello world"; const words = str.split(" ");
Common causes of the “.split is not a function” error
Calling split on a non-string
Attempting to use split
on data types other than a string will cause this error:
const num = 100; const splitNum = num.split(","); // TypeError: split is not a function
Undefined or null values
If the variable is undefined
or null
, calling split
will throw an error:
let value; const result = value.split(","); // TypeError: Cannot read property 'split' of undefined
Non-string objects
Objects that are not strings, even if they have a string-like structure, won't have the split
method:
const obj = { value: "hello world" }; const splitObj = obj.split(" "); // TypeError: split is not a function
How to diagnose the issue
Check the data type
Before calling split
, ensure the variable is a string:
const value = getSomeValue(); if (typeof value === 'string') { const result = value.split(","); }
Handle null or undefined
Make sure to handle cases where the value could be null
or undefined
:
const value = getValueThatMayBeNull(); if (value) { const result = value.split(","); }
Convert non-string values
If you have a number or a similar value, convert it to a string first:
const num = 100; const splitNum = num.toString().split(",");
Using split with arrays and objects
Arrays
If you're dealing with an array and looking to concatenate its elements, you might think of using split
, but join
is the correct method:
const arr = ['hello', 'world']; const str = arr.join(" "); // 'hello world'
Objects
For objects, you may want to serialize them to JSON or extract string properties before splitting:
const obj = { value: "hello world" }; const splitObj = obj.value.split(" ");
Handling edge cases
Coercible objects
When working with objects that have a toString
method, you can call this method to obtain a string before splitting:
const objWithToString = { toString() { return "hello world"; } }; const splitObjWithToString = objWithToString.toString().split(" ");
Exotic primitives
When dealing with Symbol or other non-string primitive types that cannot be converted to a string, ensure you're not trying to split them:
const sym = Symbol("mySymbol"); // This will throw an error because symbols cannot be converted to a string implicitly const splitSymbol = sym.split(",");
Ensuring method existence
Confirm that the split
method exists on the string object and has not been overridden or deleted:
if (typeof String.prototype.split !== 'undefined') { // split method exists and can be used }
Use of TypeScript or JSDoc for safer coding
Using TypeScript adds a layer of type safety, catching potential errors at compile-time:
function splitString(input: string) { return input.split(","); }
Similarly, JSDoc helps in type-checking within JavaScript:
/** * @param {string} input */ function splitString(input) { return input.split(","); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Integration with linting tools
Linting tools like ESLint can statically analyze your code and catch errors where non-string values are passed to split
:
{ "rules": { "no-implicit-coercion": ["error", { "allow": ["!!", "~"], "disallowTemplateShorthand": true }] } }
Handling user input
Sanitize and validate user input to avoid unexpected types being passed to split
:
const userInput = getUserInput(); if (typeof userInput === 'string') { const result = userInput.split(","); }
Performance considerations
Be cautious about using split
in performance-critical code, especially within loops, as creating many small strings and arrays can be costly.
Debugging techniques
Utilize console.log
or debugging tools to check variables' types before the split
call:
console.log(typeof value); // Should be 'string' before calling split
Polyfills for older environments
For extremely old JavaScript environments lacking split
, a polyfill can be implemented:
if (!String.prototype.split) { // Provide a polyfill for split }
Code examples in different contexts
Show usage in different environments, adjusting for framework-specific patterns and best practices.
Testing your fix
After implementing a fix, ensure to write comprehensive unit tests that cover a variety of inputs, including edge cases, to guarantee the robustness of your solution.
TOC
November 7, 2023
"split is not a function" is an error thrown in JavaScript when the split
method is called on a value that is not a string. If you keep getting “split is not a function,” keep reading. This guide walks you through why this error occurs and how to resolve it.
Understanding the split function
The split()
method in JavaScript is used to divide a string into an ordered list of substrates, puts these substrates into an array, and returns the array. It's important to note that split()
is a string method:
const str = "hello world"; const words = str.split(" ");
Common causes of the “.split is not a function” error
Calling split on a non-string
Attempting to use split
on data types other than a string will cause this error:
const num = 100; const splitNum = num.split(","); // TypeError: split is not a function
Undefined or null values
If the variable is undefined
or null
, calling split
will throw an error:
let value; const result = value.split(","); // TypeError: Cannot read property 'split' of undefined
Non-string objects
Objects that are not strings, even if they have a string-like structure, won't have the split
method:
const obj = { value: "hello world" }; const splitObj = obj.split(" "); // TypeError: split is not a function
How to diagnose the issue
Check the data type
Before calling split
, ensure the variable is a string:
const value = getSomeValue(); if (typeof value === 'string') { const result = value.split(","); }
Handle null or undefined
Make sure to handle cases where the value could be null
or undefined
:
const value = getValueThatMayBeNull(); if (value) { const result = value.split(","); }
Convert non-string values
If you have a number or a similar value, convert it to a string first:
const num = 100; const splitNum = num.toString().split(",");
Using split with arrays and objects
Arrays
If you're dealing with an array and looking to concatenate its elements, you might think of using split
, but join
is the correct method:
const arr = ['hello', 'world']; const str = arr.join(" "); // 'hello world'
Objects
For objects, you may want to serialize them to JSON or extract string properties before splitting:
const obj = { value: "hello world" }; const splitObj = obj.value.split(" ");
Handling edge cases
Coercible objects
When working with objects that have a toString
method, you can call this method to obtain a string before splitting:
const objWithToString = { toString() { return "hello world"; } }; const splitObjWithToString = objWithToString.toString().split(" ");
Exotic primitives
When dealing with Symbol or other non-string primitive types that cannot be converted to a string, ensure you're not trying to split them:
const sym = Symbol("mySymbol"); // This will throw an error because symbols cannot be converted to a string implicitly const splitSymbol = sym.split(",");
Ensuring method existence
Confirm that the split
method exists on the string object and has not been overridden or deleted:
if (typeof String.prototype.split !== 'undefined') { // split method exists and can be used }
Use of TypeScript or JSDoc for safer coding
Using TypeScript adds a layer of type safety, catching potential errors at compile-time:
function splitString(input: string) { return input.split(","); }
Similarly, JSDoc helps in type-checking within JavaScript:
/** * @param {string} input */ function splitString(input) { return input.split(","); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Integration with linting tools
Linting tools like ESLint can statically analyze your code and catch errors where non-string values are passed to split
:
{ "rules": { "no-implicit-coercion": ["error", { "allow": ["!!", "~"], "disallowTemplateShorthand": true }] } }
Handling user input
Sanitize and validate user input to avoid unexpected types being passed to split
:
const userInput = getUserInput(); if (typeof userInput === 'string') { const result = userInput.split(","); }
Performance considerations
Be cautious about using split
in performance-critical code, especially within loops, as creating many small strings and arrays can be costly.
Debugging techniques
Utilize console.log
or debugging tools to check variables' types before the split
call:
console.log(typeof value); // Should be 'string' before calling split
Polyfills for older environments
For extremely old JavaScript environments lacking split
, a polyfill can be implemented:
if (!String.prototype.split) { // Provide a polyfill for split }
Code examples in different contexts
Show usage in different environments, adjusting for framework-specific patterns and best practices.
Testing your fix
After implementing a fix, ensure to write comprehensive unit tests that cover a variety of inputs, including edge cases, to guarantee the robustness of your solution.
November 7, 2023
"split is not a function" is an error thrown in JavaScript when the split
method is called on a value that is not a string. If you keep getting “split is not a function,” keep reading. This guide walks you through why this error occurs and how to resolve it.
Understanding the split function
The split()
method in JavaScript is used to divide a string into an ordered list of substrates, puts these substrates into an array, and returns the array. It's important to note that split()
is a string method:
const str = "hello world"; const words = str.split(" ");
Common causes of the “.split is not a function” error
Calling split on a non-string
Attempting to use split
on data types other than a string will cause this error:
const num = 100; const splitNum = num.split(","); // TypeError: split is not a function
Undefined or null values
If the variable is undefined
or null
, calling split
will throw an error:
let value; const result = value.split(","); // TypeError: Cannot read property 'split' of undefined
Non-string objects
Objects that are not strings, even if they have a string-like structure, won't have the split
method:
const obj = { value: "hello world" }; const splitObj = obj.split(" "); // TypeError: split is not a function
How to diagnose the issue
Check the data type
Before calling split
, ensure the variable is a string:
const value = getSomeValue(); if (typeof value === 'string') { const result = value.split(","); }
Handle null or undefined
Make sure to handle cases where the value could be null
or undefined
:
const value = getValueThatMayBeNull(); if (value) { const result = value.split(","); }
Convert non-string values
If you have a number or a similar value, convert it to a string first:
const num = 100; const splitNum = num.toString().split(",");
Using split with arrays and objects
Arrays
If you're dealing with an array and looking to concatenate its elements, you might think of using split
, but join
is the correct method:
const arr = ['hello', 'world']; const str = arr.join(" "); // 'hello world'
Objects
For objects, you may want to serialize them to JSON or extract string properties before splitting:
const obj = { value: "hello world" }; const splitObj = obj.value.split(" ");
Handling edge cases
Coercible objects
When working with objects that have a toString
method, you can call this method to obtain a string before splitting:
const objWithToString = { toString() { return "hello world"; } }; const splitObjWithToString = objWithToString.toString().split(" ");
Exotic primitives
When dealing with Symbol or other non-string primitive types that cannot be converted to a string, ensure you're not trying to split them:
const sym = Symbol("mySymbol"); // This will throw an error because symbols cannot be converted to a string implicitly const splitSymbol = sym.split(",");
Ensuring method existence
Confirm that the split
method exists on the string object and has not been overridden or deleted:
if (typeof String.prototype.split !== 'undefined') { // split method exists and can be used }
Use of TypeScript or JSDoc for safer coding
Using TypeScript adds a layer of type safety, catching potential errors at compile-time:
function splitString(input: string) { return input.split(","); }
Similarly, JSDoc helps in type-checking within JavaScript:
/** * @param {string} input */ function splitString(input) { return input.split(","); }
You could ship faster.
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.

Integration with linting tools
Linting tools like ESLint can statically analyze your code and catch errors where non-string values are passed to split
:
{ "rules": { "no-implicit-coercion": ["error", { "allow": ["!!", "~"], "disallowTemplateShorthand": true }] } }
Handling user input
Sanitize and validate user input to avoid unexpected types being passed to split
:
const userInput = getUserInput(); if (typeof userInput === 'string') { const result = userInput.split(","); }
Performance considerations
Be cautious about using split
in performance-critical code, especially within loops, as creating many small strings and arrays can be costly.
Debugging techniques
Utilize console.log
or debugging tools to check variables' types before the split
call:
console.log(typeof value); // Should be 'string' before calling split
Polyfills for older environments
For extremely old JavaScript environments lacking split
, a polyfill can be implemented:
if (!String.prototype.split) { // Provide a polyfill for split }
Code examples in different contexts
Show usage in different environments, adjusting for framework-specific patterns and best practices.
Testing your fix
After implementing a fix, ensure to write comprehensive unit tests that cover a variety of inputs, including edge cases, to guarantee the robustness of your solution.
What is Basedash?
What is Basedash?
What is Basedash?
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.




Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
USER CRM
ADMIN PANEL
SQL COMPOSER WITH AI

Related posts
Related posts
Related posts



How to Remove Characters from a String in JavaScript
Jeremy Sarchet



How to Sort Strings in JavaScript
Max Musing



How to Remove Spaces from a String in JavaScript
Jeremy Sarchet



Detecting Prime Numbers in JavaScript
Robert Cooper



How to Parse Boolean Values in JavaScript
Max Musing



How to Remove a Substring from a String in JavaScript
Robert Cooper