
How to move a JavaScript element
You can move a JavaScript element within the DOM (Document Object Model) in several ways. We’ll cover them in this guide.
Understand the DOM structure
Before moving an element, it's essential to understand its position within the DOM. JavaScript provides access to the DOM tree, allowing you to manipulate elements by selecting them and altering their position.
const element = document.getElementById('elementId');
Select the element to move
To move an element, you must first select it. This can be done using document.getElementById
, document.querySelector
, or similar methods.
const elementToMove = document.querySelector('.element-class');
Move using DOM manipulation methods
Append to a new parent
You can move an element by appending it to another parent element. This will move the element to the end of the new parent.
const newParent = document.querySelector('.new-parent-class'); newParent.appendChild(elementToMove);
Insert before another element
To place an element at a specific point in the DOM, use parentNode.insertBefore
.
const referenceElement = document.querySelector('.reference-class'); referenceElement.parentNode.insertBefore(elementToMove, referenceElement);
Replace an existing element
If you want to swap an element with another, use parentNode.replaceChild
.
const elementToReplace = document.querySelector('.replace-class'); elementToReplace.parentNode.replaceChild(elementToMove, elementToReplace);
Move using CSS manipulation
Change position properties
For visual movement without altering the DOM structure, CSS properties like top
, left
, right
, and bottom
can be used along with position
.
elementToMove.style.position = 'absolute'; elementToMove.style.left = '100px'; elementToMove.style.top = '50px';
Use CSS classes
You can also define CSS classes for different positions and toggle them with JavaScript.
.moved-element { position: absolute; top: 50px; left: 100px; }
elementToMove.classList.add('moved-element');
Implement drag and drop functionality
For interactive movement, you might implement drag and drop using mouse events.
Add event listeners
elementToMove.addEventListener('mousedown', mouseDownHandler); document.addEventListener('mouseup', mouseUpHandler); document.addEventListener('mousemove', mouseMoveHandler);
Implement event handlers
let offsetX, offsetY, drag = false; function mouseDownHandler(e) { drag = true; offsetX = e.clientX - elementToMove.getBoundingClientRect().left; offsetY = e.clientY - elementToMove.getBoundingClientRect().top; document.addEventListener('mousemove', mouseMoveHandler); } function mouseUpHandler() { drag = false; document.removeEventListener('mousemove', mouseMoveHandler); } function mouseMoveHandler(e) { if (drag) { elementToMove.style.left = `${e.clientX - offsetX}px`; elementToMove.style.top = `${e.clientY - offsetY}px`; } }
Enhance with animations and transitions
Adding CSS transitions can make the movement of elements smoother and visually appealing.
elementToMove.style.transition = 'all 0.5s ease'; // Change the position afterward elementToMove.style.left = '200px';
Handle touch events for mobile devices
For mobile compatibility, you can manage touch events similarly to mouse events for drag and drop.
elementToMove.addEventListener('touchstart', touchStartHandler); elementToMove.addEventListener('touchmove', touchMoveHandler); elementToMove.addEventListener('touchend', touchEndHandler);
Handle dynamic content
If the element or its destination is dynamically generated, ensure the code runs after the elements are available in the DOM. Event delegation or MutationObservers can be used for handling such situations.
Address potential performance issues
Consider the performance implications of moving elements. Use requestAnimationFrame
for animations and be mindful of layout thrashing when manipulating the DOM.
Use JavaScript frameworks and libraries
Frameworks like jQuery, React, Vue, or Angular provide their own syntax and methods for moving elements, which can simplify the process.
Cloning elements
In some cases, you might want to move an element by creating a clone and inserting it elsewhere in the DOM.
const clone = elementToMove.cloneNode(true); newParent.appendChild(clone);
Invite only
We're building the next generation of data visualization.
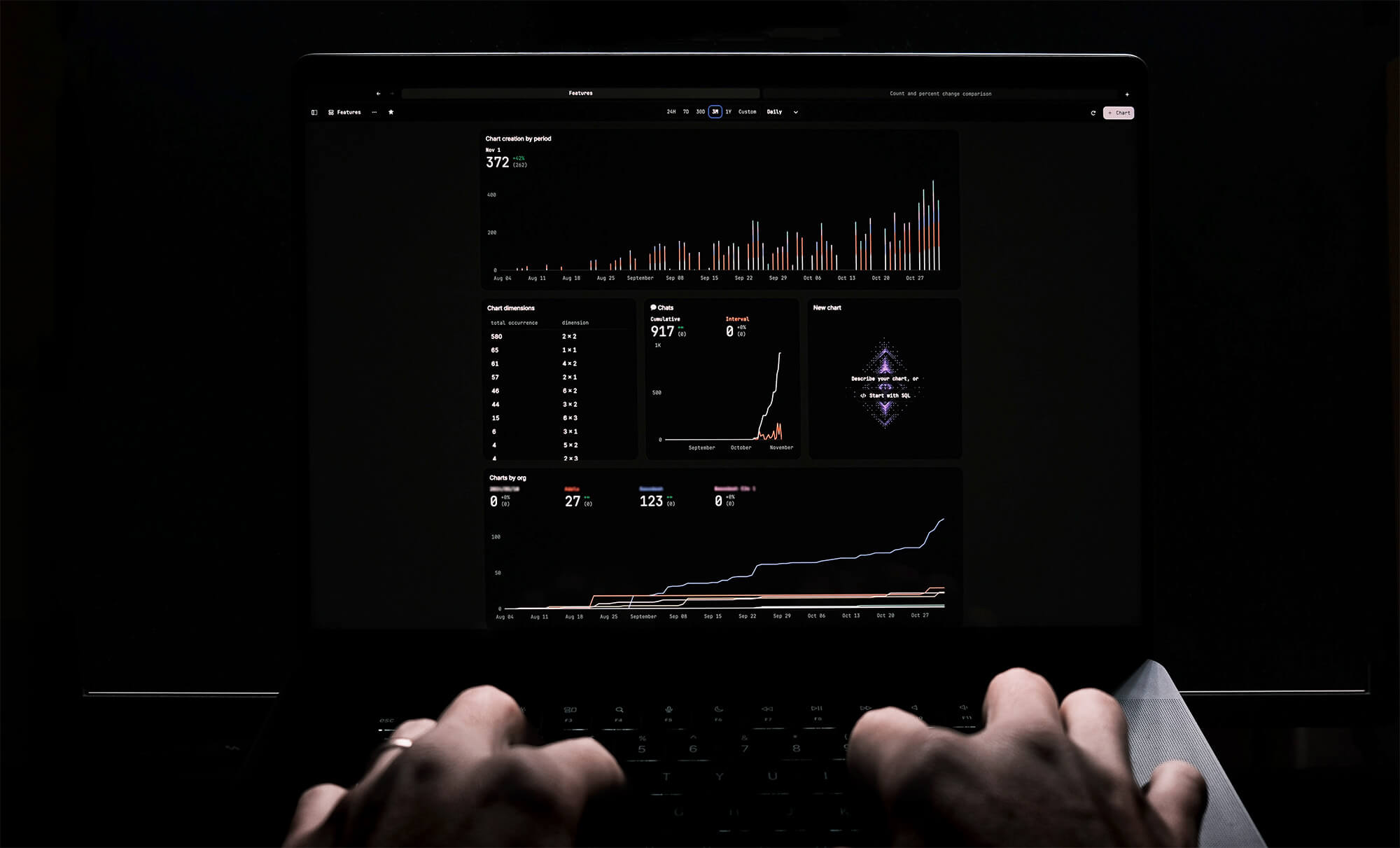
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper