
How to remove an element from a set in JavaScript
Removing an element from a set in JavaScript can be accomplished with the delete
method provided by the Set
object. This method efficiently eliminates the specified element from a set without needing to recreate the set or manually iterate over its elements.
Understanding the Set object
A Set
is a collection of unique values; no value can occur more than once. The Set
object provides simple and straightforward methods to manage its contents, including adding, deleting, and checking for the presence of elements.
Remove an element using delete
The delete
method is specifically designed to remove an element from a set. If the element specified is present in the set, delete
will remove it and return true
; otherwise, it will return false
.
Here's an example of how to use delete
:
let mySet = new Set([1, 2, 3, 4, 5]); mySet.delete(3); // Removes the element '3' from the set console.log(mySet.has(3)); // false, as 3 has been removed
Check if an element is present before deleting
While the delete
method will fail silently by returning false
if an element isn't found, you may want to check if an element exists before attempting to delete it.
let mySet = new Set([1, 2, 3, 4, 5]); if (mySet.has(3)) { mySet.delete(3); console.log('Element 3 was removed.'); } else { console.log('Element not found in the set.'); }
Deleting non-existent elements
Trying to remove a non-existent element won't affect the set or cause an error.
let mySet = new Set([1, 2, 3]); mySet.delete(4); // Returns false, mySet remains unchanged
Handling complex data types
Set
objects can store complex data types like objects and arrays. When deleting such elements, the reference must match exactly.
let obj = { key: 'value' }; let mySet = new Set([obj]); // To remove the object, you must use the exact reference mySet.delete(obj); // The object is removed from the set
Clearing a set
If the goal is to remove all elements from a set, the clear
method is the appropriate choice.
let mySet = new Set([1, 2, 3, 4, 5]); mySet.clear(); // Removes all elements from the set console.log(mySet.size); // 0
Performance considerations when removing a JavaScript set
The delete
method operates in constant time (O(1)) for the Set
object, meaning that the operation takes the same amount of time regardless of the size of the set.
Browser compatibility
The delete
method is widely supported in modern browsers, but for older browsers like Internet Explorer (version 11 and below), a polyfill or alternative solution might be necessary. Always check compatibility if the script is intended for use in environments with older browsers.
Invite only
We're building the next generation of data visualization.
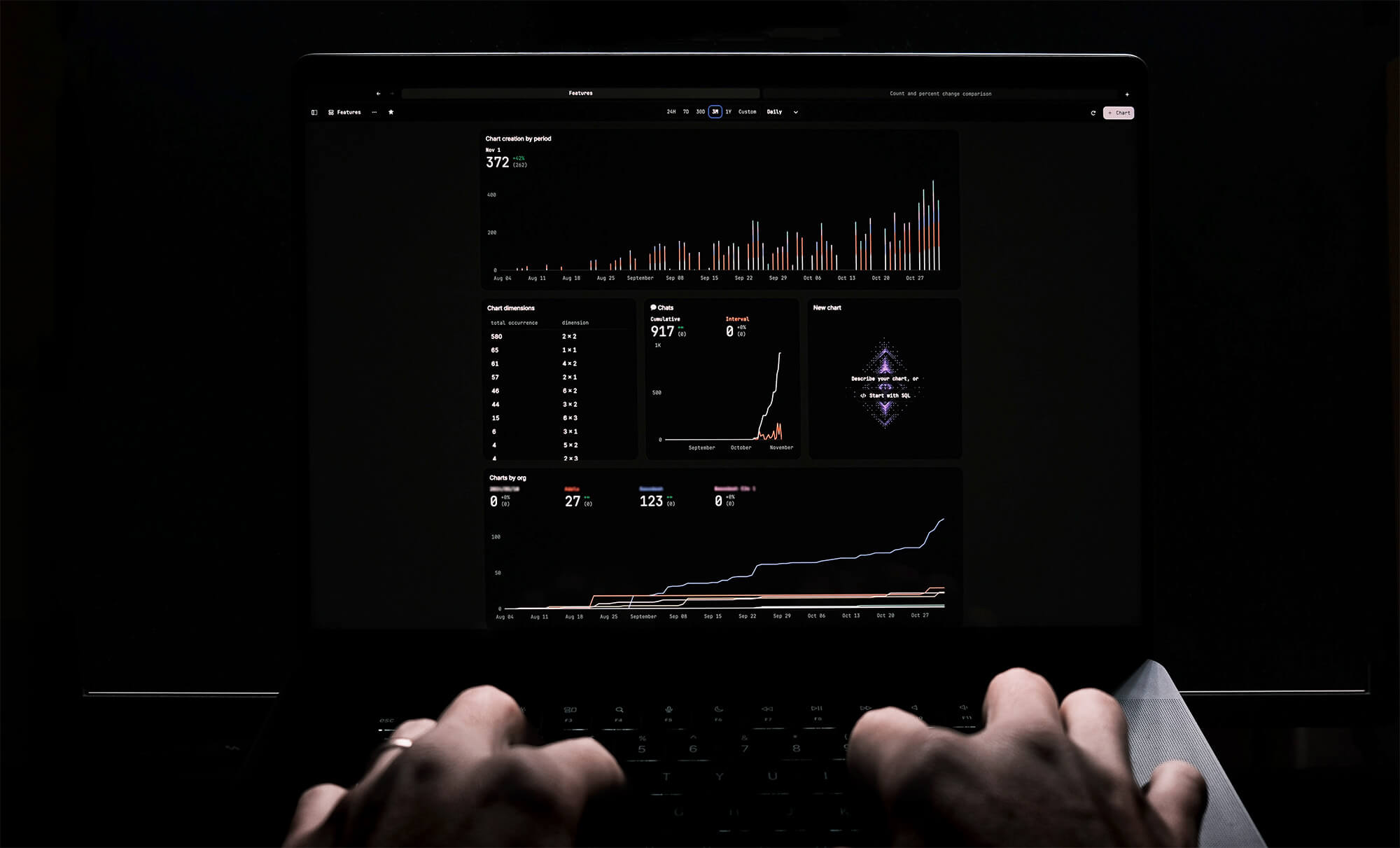
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper