
How to set the cursor position in JavaScript
To set the cursor position in JavaScript you’ll need to manipulate the caret within an input field or a contenteditable element. In this guide we’ll cover how to control the cursor's location to improve user experience and interactivity in web apps.
Understanding the DOM elements
Before manipulating the cursor position, it’s essential to understand that the operation is typically performed on text-based input elements (<input>
, <textarea>
) or elements with the contenteditable
attribute set to true.
Fetching the DOM element
To set the cursor position, first select the DOM element using methods like getElementById()
or querySelector()
.
let inputElement = document.getElementById('myInput'); // or let editableElement = document.querySelector('[contenteditable]');
Using selection and range APIs
setSelectionRange
for input elements
For <input>
or <textarea>
elements, you can use the setSelectionRange
method to place the cursor at a specific index.
inputElement.setSelectionRange(5, 5); // Places the cursor at the 5th index
This method also allows you to select a range of text by providing different start and end indexes.
createRange
and addRange
for contenteditable elements
For elements with contenteditable
, the Range
and Selection
APIs come into play.
let range = document.createRange(); let sel = window.getSelection();
Setting cursor position
For input elements
function setCursorPosition(inputElem, position) { if (inputElem.setSelectionRange) { inputElem.focus(); inputElem.setSelectionRange(position, position); } }
For contenteditable elements
function setCursorEditable(editableElem, position) { let range = document.createRange(); let sel = window.getSelection(); range.setStart(editableElem.childNodes[0], position); range.collapse(true); sel.removeAllRanges(); sel.addRange(range); editableElem.focus(); }
Dealing with line breaks and nodes
For multi-line content or content with multiple child nodes, you’ll need to traverse the nodes and count their lengths to position the cursor correctly.
function setCursorAtNodePosition(node, index) { let range = document.createRange(); let selection = window.getSelection(); let currentPos = 0; let found = false; function searchNode(node) { if (node.nodeType === Node.TEXT_NODE) { if (currentPos + node.length >= index) { range.setStart(node, index - currentPos); range.collapse(true); found = true; } else { currentPos += node.length; } } else { for (let child of node.childNodes) { if (found) break; searchNode(child); } } } searchNode(node); selection.removeAllRanges(); selection.addRange(range); }
Handling cursor position on focus
It's common to set the cursor position when the element gains focus.
inputElement.addEventListener('focus', () => { setCursorPosition(inputElement, 0); // places the cursor at the beginning });
Cross-browser considerations
While modern browsers support these methods, always test across different browsers to ensure compatibility. For older versions of IE, you might need to use createTextRange()
instead.
if (document.selection) { // For IE let range = inputElement.createTextRange(); range.move('character', position); range.select(); }
Cursor position in editable content with formatting
For complex contenteditable elements with formatting, you may need to account for HTML tags when calculating positions.
Considerations for dynamic content
When content changes dynamically, cursor position may need to be recalculated. Watch for changes and adjust the cursor position accordingly.
Invite only
We're building the next generation of data visualization.
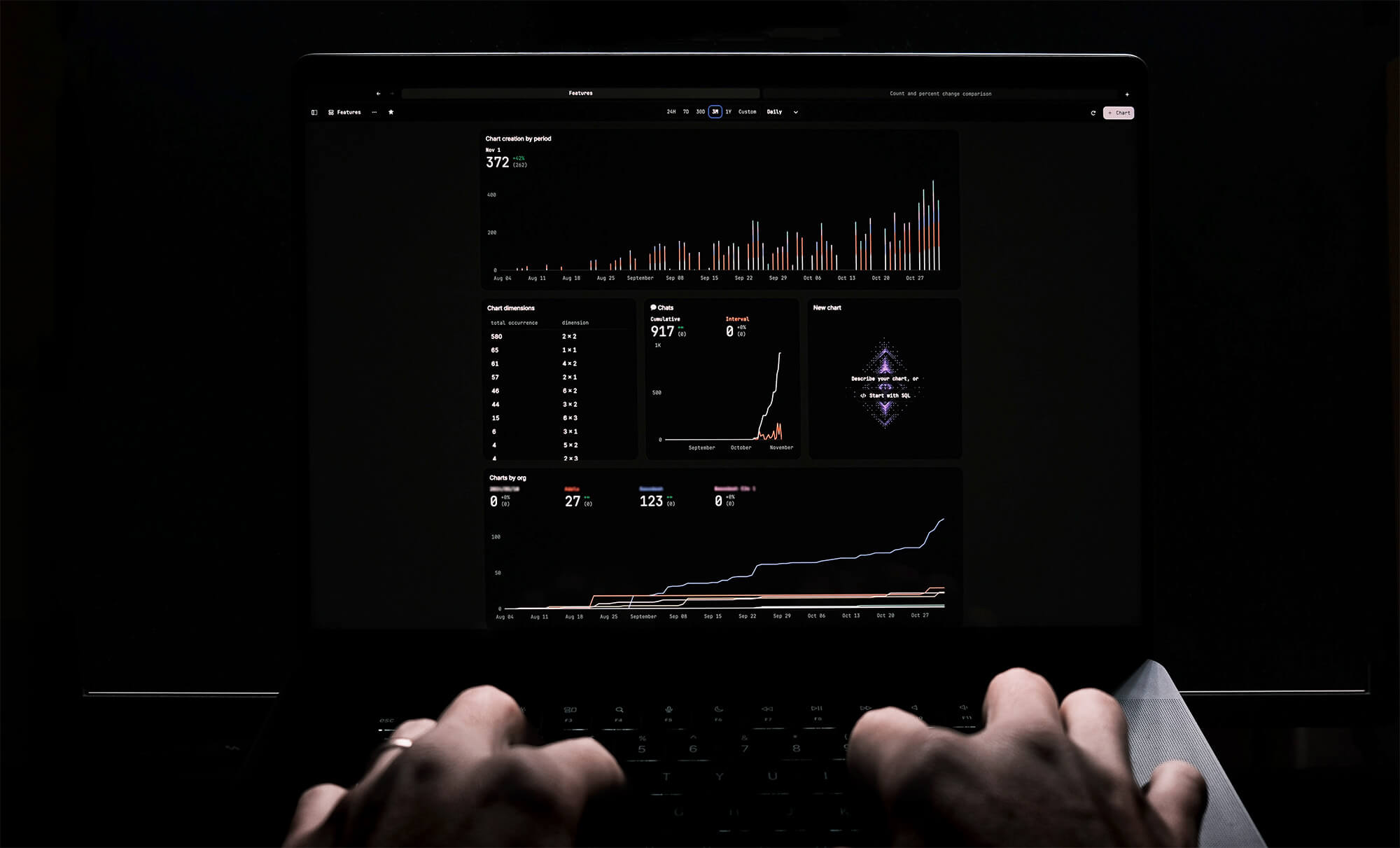
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper