
JavaScript Cannot Set Property of Undefined
Attempting to set a property on undefined
indicates that the code is trying to use a variable that hasn't been initialized. This guide explores common causes and solutions for this error, helping engineers quickly identify and rectify the issue.
Understanding the Error
The error "Cannot set property of 'X' of undefined" occurs in JavaScript when you attempt to assign a value to a property of an undefined variable. This typically happens when an object is expected, but the variable is either not declared, not initialized, or does not have an assigned value.
Common Causes
Variable Has Not Been Defined
If a variable is used before it has been declared, JavaScript will throw an error.
let user; user.name = "Alice"; // Error: Cannot set property 'name' of undefined
Function Does Not Return Value
A function without a return statement, or with an empty return, returns undefined
by default.
function createUser() { // no return statement } const user = createUser(); user.name = "Alice"; // Error
Incorrect Object Path
Attempting to access a deeply nested property without ensuring each level exists can lead to the error.
const user = {}; user.profile.details.name = "Alice"; // Error
Solutions
Initialize Your Variables
Always initialize variables before using them.
let user = {}; // Initialize as an empty object user.name = "Alice";
Ensure Functions Return Objects
Make sure your functions return an object if you need to set properties on their output.
function createUser() { return {}; } const user = createUser(); user.name = "Alice";
Use Optional Chaining
Optional chaining (?.
) allows you to safely access deeply nested properties.
const user = {}; user.profile?.details.name = "Alice"; // No error, but won't set 'name'
Guard Clauses
Check if an object exists before setting its properties.
if (user) { user.name = "Alice"; }
Debugging Tips
Use console.log
Print the variable to the console before the error occurs to inspect its value.
console.log(user); // Check if 'user' is undefined
Linters and IDEs
Use a linter or an IDE that can catch these errors before runtime.
Testing
Write tests to verify that your functions always return the expected values.
Conclusion
By initializing variables, ensuring functions return values, correctly referencing object paths, and employing defensive programming techniques, you can prevent and fix the "Cannot set property of 'X' of undefined" error in your JavaScript code.
Invite only
We're building the next generation of data visualization.
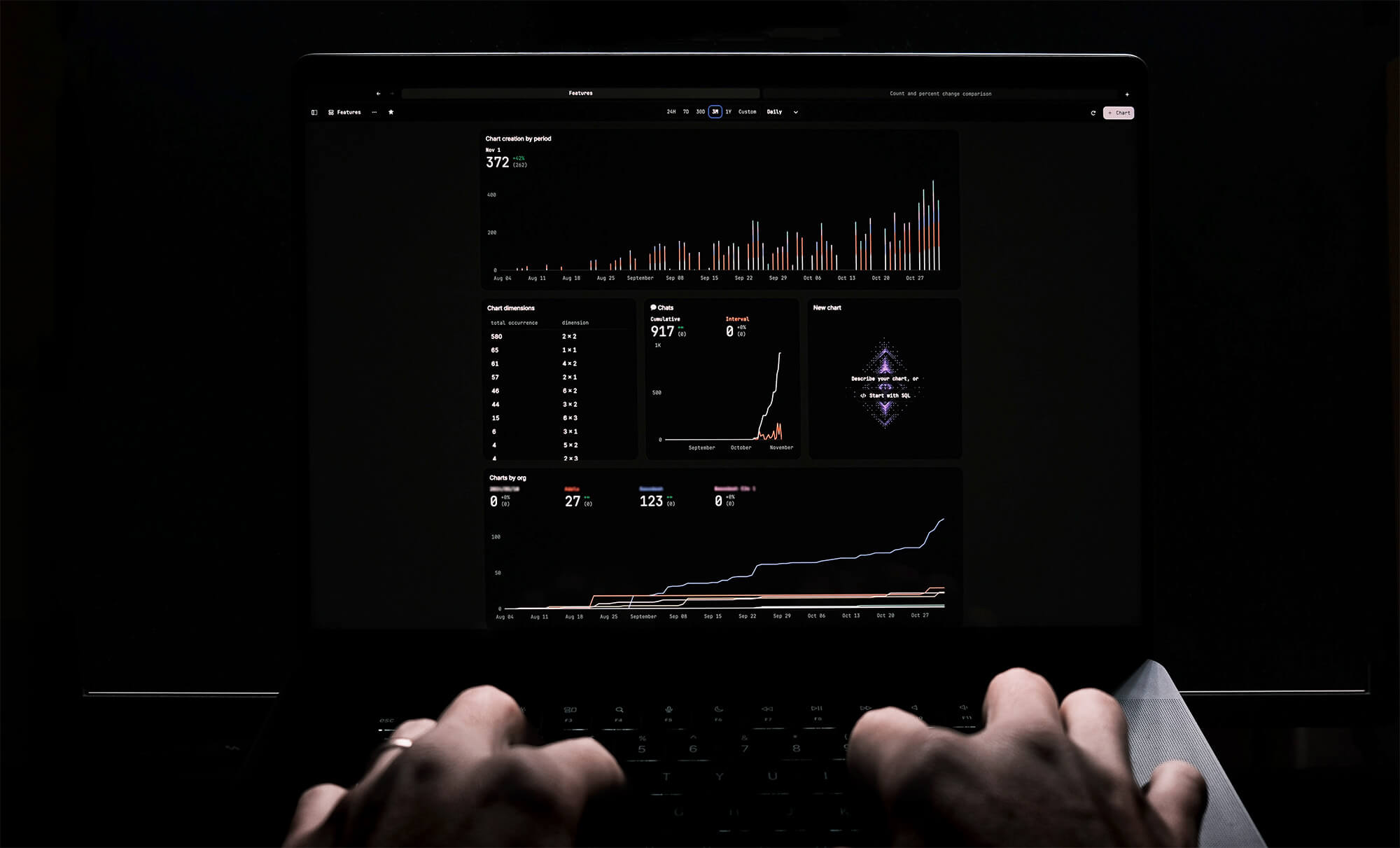
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper