
JavaScript String Format Techniques
JavaScript offers various methods to format strings. This guide covers the most common JavaScript techniques to format a string: template literals, concatenation, and the Intl
object for locale-specific formatting.
How to use template literals in JavaScript?
Template literals, introduced in ES6, provide an easy way to create formatted JavaScript strings. They allow for embedded expressions, which are enclosed by the dollar sign and curly braces (${expression}
), and are enclosed by backticks (`).
const name = "Jane"; const greeting = `Hello, ${name}!`; console.log(greeting); // Output: Hello, Jane!
How to concatenate strings in JS?
Before template literals, string concatenation with the +
operator was commonly used. This method is still viable, especially for simple concatenations.
const firstName = "John"; const lastName = "Doe"; const fullName = firstName + " " + lastName; console.log(fullName); // Output: John Doe
How to format numbers in JS?
For formatting numbers, the Intl.NumberFormat
object provides locale-sensitive number formatting.
const number = 123456.789; // German uses comma as decimal separator and period for thousands const formattedNumber = new Intl.NumberFormat('de-DE').format(number); console.log(formattedNumber); // Output: 123.456,789
How to format dates in JS?
Similarly, the Intl.DateTimeFormat
object allows for the formatting of dates in a locale-sensitive manner.
const date = new Date(); // British English uses day-month-year format const formattedDate = new Intl.DateTimeFormat('en-GB').format(date); console.log(formattedDate); // Output: 20/04/2023
Custom formatting functions
For more complex or specific formatting needs, creating custom functions is a practical solution.
function formatCurrency(value, locale = 'en-US', currency = 'USD') { return new Intl.NumberFormat(locale, { style: 'currency', currency: currency }).format(value); } const price = 999.99; console.log(formatCurrency(price)); // Output: $999.99
Invite only
We're building the next generation of data visualization.
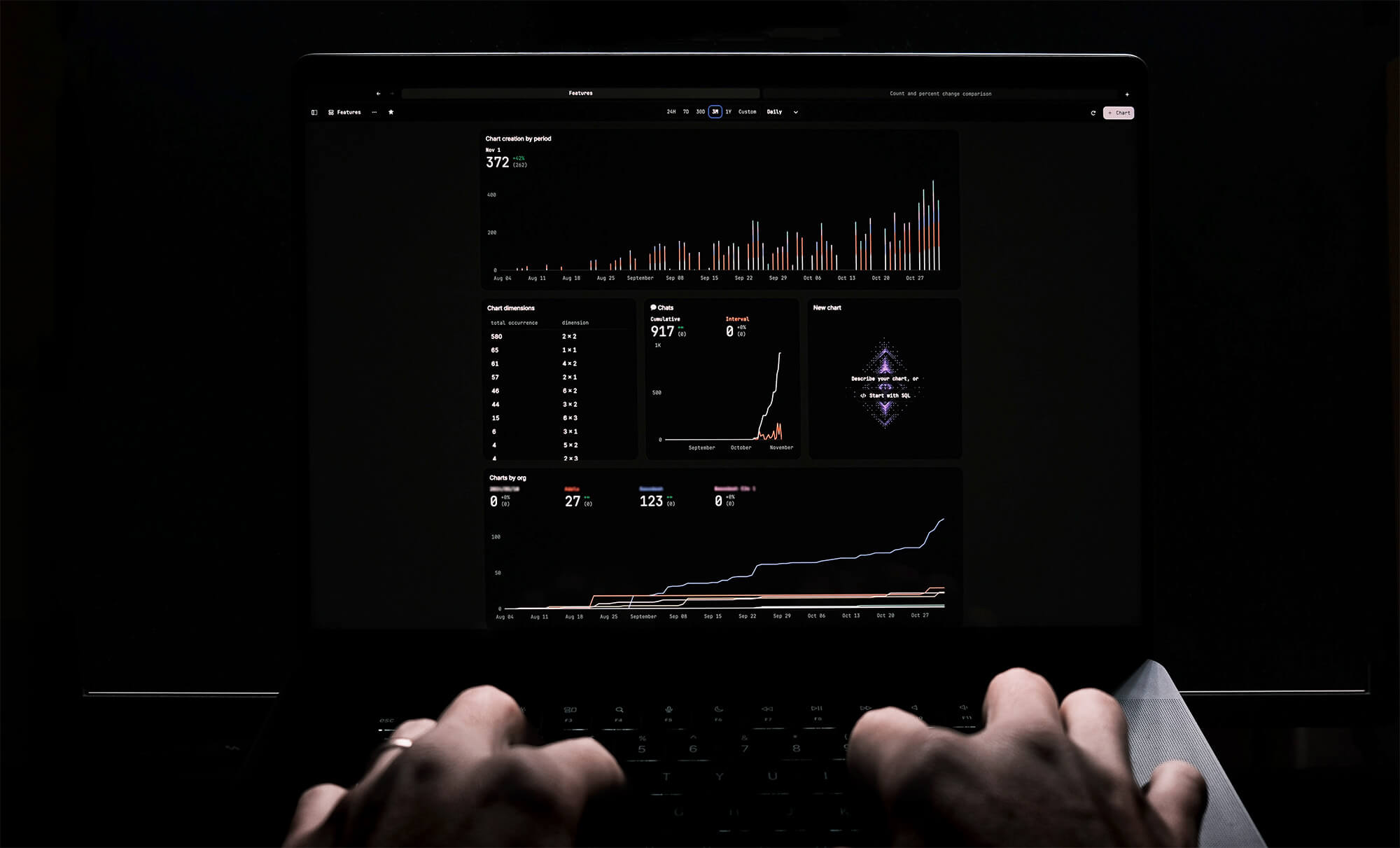
How to Remove Characters from a String in JavaScript
Jeremy Sarchet
How to Sort Strings in JavaScript
Max Musing
How to Remove Spaces from a String in JavaScript
Jeremy Sarchet
Detecting Prime Numbers in JavaScript
Robert Cooper
How to Parse Boolean Values in JavaScript
Max Musing
How to Remove a Substring from a String in JavaScript
Robert Cooper