
MongoDB CRUD operations in Python
MongoDB, a popular NoSQL database, is widely used in numerous applications due to its scalability and flexibility.
Python developers often interface with MongoDB using a package called pymongo
. This guide will walk you through the basic CRUD (Create, Read, Update, Delete) operations in MongoDB using Python.
Prerequisites
- MongoDB installed on your system
- Python environment set up
- pymongo installed (
pip install pymongo
)
1. Establishing a Connection
First, you need to connect to your MongoDB instance:
from pymongo import MongoClient # Connect to the default host and port client = MongoClient() # Alternatively, specify the host and port # client = MongoClient('localhost', 27017)
2. Database and Collection
MongoDB organizes data in databases and collections. Here's how to specify them:
# Choose a database (it will be created if it doesn’t exist) db = client.mydatabase # Choose a collection (similar to a table in relational databases) collection = db.mycollection
3. CRUD Operations
Create
To insert a document into a collection:
doc = {"name": "John", "age": 30, "city": "New York"} result = collection.insert_one(doc) print(result.inserted_id)
For multiple documents:
docs = [ {"name": "Anna", "age": 25, "city": "London"}, {"name": "Mike", "age": 32, "city": "San Francisco"} ] result = collection.insert_many(docs) print(result.inserted_ids)
Read
To retrieve one document:
# Find one document without any criteria result = collection.find_one() print(result) # Find one document with a criteria result = collection.find_one({"name": "John"}) print(result)
To retrieve multiple documents:
# Find all documents results = collection.find() # Find documents with criteria results = collection.find({"age": {"$gt": 30}}) for r in results: print(r)
Update
To update one document:
# Update the age of John criteria = {"name": "John"} new_values = {"$set": {"age": 31}} collection.update_one(criteria, new_values)
To update multiple documents:
# Increase age by 1 for all documents where age is less than 30 criteria = {"age": {"$lt": 30}} new_values = {"$inc": {"age": 1}} collection.update_many(criteria, new_values)
Delete
To delete one document:
# Delete a document with name John collection.delete_one({"name": "John"})
To delete multiple documents:
# Delete all documents where age is greater than 30 collection.delete_many({"age": {"$gt": 30}})
4. Closing the Connection
After performing your operations, it's good practice to close the connection:
client.close()
Conclusion
MongoDB CRUD operations in Python using pymongo
are straightforward. This guide covers the basics to get you started. However, MongoDB offers more advanced features such as indexing, aggregation, and transactions, which are worth exploring as you dive deeper into MongoDB and Python development.
Invite only
We're building the next generation of data visualization.
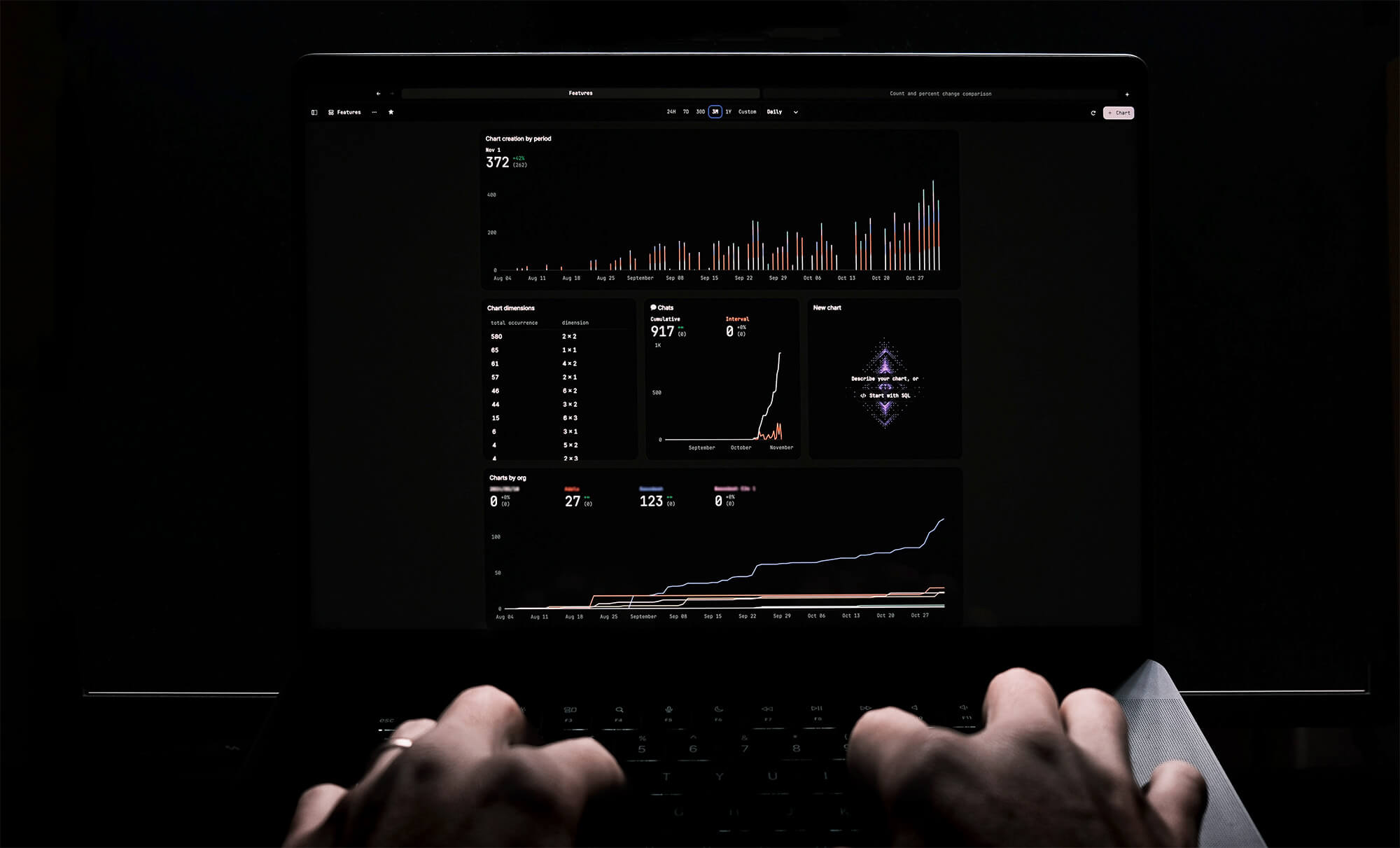
How to Center a Table in HTML with CSS
Jeremy Sarchet
Adjusting HTML Table Column Width for Better Design
Robert Cooper
How to Link Multiple CSS Stylesheets in HTML
Robert Cooper
Mastering HTML Table Inline Styling: A Guide
Max Musing
HTML Multiple Style Attributes: A Quick Guide
Max Musing
How to Set HTML Table Width for Responsive Design
Max Musing