
onSuccess in React Query: A Guide
React Query's onSuccess
callback is a great way to handle data fetching in a React application. It triggers when a query successfully fetches data, allowing developers to perform side effects or update the UI accordingly.
What is onSuccess
in React Query?
onSuccess
is a callback function that you can define within a query or mutation in React Query. This function is executed when the query or mutation successfully retrieves data or completes an action without errors. The primary use of onSuccess
is to perform additional actions or side effects, like updating local state, triggering notifications, or redirecting the user.
How to use onSuccess in queries
When using React Query's useQuery
or similar hooks, onSuccess
can be passed as an option. It receives the data returned from the query as its argument.
import { useQuery } from 'react-query'; const fetchData = async () => { // fetch logic }; const Component = () => { const { data } = useQuery('queryKey', fetchData, { onSuccess: (data) => { // handle successful data fetching } }); // component logic };
How to use onSuccess in mutations
For mutations, onSuccess
is used in a similar way within the useMutation
hook. It provides the response data from the mutation operation.
import { useMutation } from 'react-query'; const mutateData = async () => { // mutation logic }; const Component = () => { const mutation = useMutation(mutateData, { onSuccess: (data) => { // handle successful mutation } }); // component logic };
Real-world Scenarios
Updating local state
onSuccess
can be used to update the local state of a component based on the fetched data.
const Component = () => { const [localData, setLocalData] = useState(null); useQuery('queryKey', fetchData, { onSuccess: (data) => { setLocalData(data); } }); // component logic };
Triggering notifications
You can use onSuccess
to show success messages or notifications to the user.
const Component = () => { const query = useQuery('queryKey', fetchData, { onSuccess: () => { alert('Data fetched successfully!'); } }); // component logic };
Redirecting after mutation
After a successful mutation, you might want to redirect the user to a different page.
const Component = () => { const mutation = useMutation(mutateData, { onSuccess: () => { // redirect logic, e.g., using React Router } }); // component logic };
Advanced use cases
Accessing query or mutation Meta
You can access the query or mutation instance within the onSuccess
callback to get additional details like query key or state.
const Component = () => { const query = useQuery('queryKey', fetchData, { onSuccess: (data) => { console.log('Query Key:', query.queryKey); // additional logic } }); // component logic };
Interacting with query cache
React Query allows you to interact with the query cache in the onSuccess
callback, enabling scenarios like updating related queries based on mutation results.
const Component = () => { const queryClient = useQueryClient(); const mutation = useMutation(mutateData, { onSuccess: () => { queryClient.invalidateQueries('relatedQueryKey'); } }); // component logic };
Invite only
We're building the next generation of data visualization.
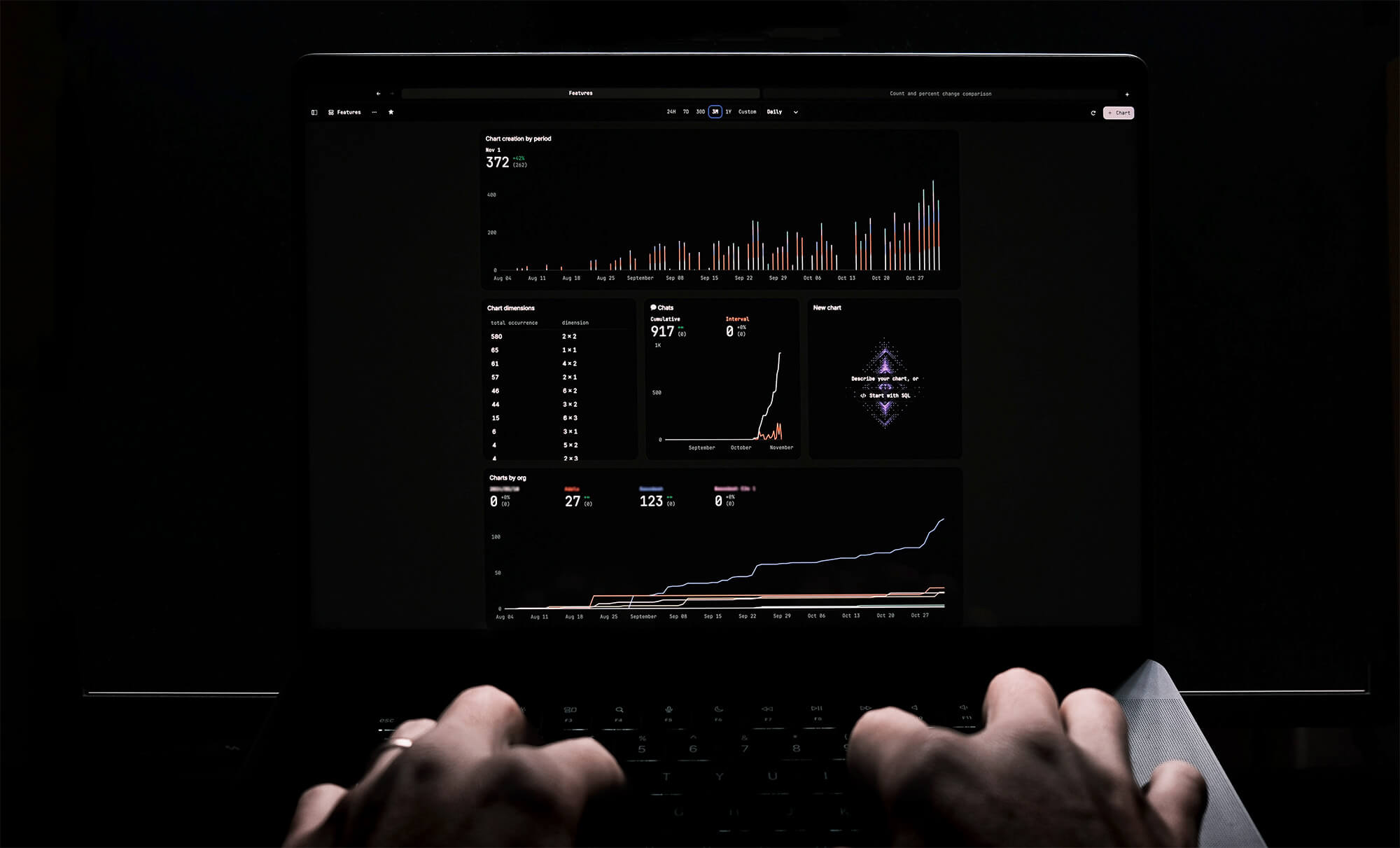
How to Center a Table in HTML with CSS
Jeremy Sarchet
Adjusting HTML Table Column Width for Better Design
Robert Cooper
How to Link Multiple CSS Stylesheets in HTML
Robert Cooper
Mastering HTML Table Inline Styling: A Guide
Max Musing
HTML Multiple Style Attributes: A Quick Guide
Max Musing
How to Set HTML Table Width for Responsive Design
Max Musing