
tRPC and React Query: Enhancing API Interactions in React Apps
Combining tRPC with React Query provides a powerful, type-safe approach to handling API requests in React applications, seamlessly integrating the type safety of tRPC with the efficiency of React Query. This guide explores how to leverage tRPC and React Query for optimized data fetching and state management.
Overview of tRPC and React Query
What is tRPC?
tRPC is a framework for building type-safe APIs in TypeScript applications. It allows developers to create APIs with minimal boilerplate, ensuring that the types defined on the server are automatically inferred in the client. This results in a more reliable and maintainable codebase.
Why Use React Query with tRPC?
React Query is a library for fetching, caching, and updating data in React applications. When used with tRPC, it provides a streamlined way to manage server state, cache data, and handle data synchronization, enhancing the overall efficiency and performance of your application.
Setting up your project
Installing dependencies
To get started, ensure you have tRPC and React Query installed in your project:
npm install @trpc/client react-query
Configuring tRPC client
Create a tRPC client instance to interact with your tRPC server:
import { createTRPCClient } from '@trpc/client'; const trpcClient = createTRPCClient({ url: '<http://localhost:3000/trpc>', });
Integrating React Query
Creating custom hooks
Custom hooks simplify data fetching and mutation operations. Define hooks for each tRPC query or mutation:
import { useQuery } from 'react-query'; export function useTRPCQuery(queryKey, queryFn, options) { return useQuery(queryKey, () => trpcClient.query(queryFn), options); }
Fetching data with queries
Utilize the custom hooks in your components to fetch data:
import React from 'react'; import { useTRPCQuery } from './hooks'; const MyComponent = () => { const { data, isLoading } = useTRPCQuery('uniqueKey', 'getSomeData'); if (isLoading) return <div>Loading...</div>; return <div>{data}</div>; };
Handling mutations
Creating a mutation hook
Similarly, create a hook for mutations:
import { useMutation } from 'react-query'; export function useTRPCMutation(mutationFn) { return useMutation((newData) => trpcClient.mutate(mutationFn, newData)); }
Using mutations in components
Implement the mutation hook in your components to update data:
import React from 'react'; import { useTRPCMutation } from './hooks'; const UpdateComponent = () => { const mutation = useTRPCMutation('updateData'); const handleSubmit = (data) => { mutation.mutate(data); }; return <form onSubmit={handleSubmit}>...</form>; };
Advanced usage
Optimistic updates
React Query supports optimistic updates, allowing the UI to update immediately before the mutation is confirmed by the server. This can be configured in the mutation options.
Query invalidation
To keep data fresh, you can invalidate queries after a mutation, triggering a refetch of related data.
Prefetching data
Prefetching data for anticipated requests can improve user experience by loading data before it's needed.
Invite only
We're building the next generation of data visualization.
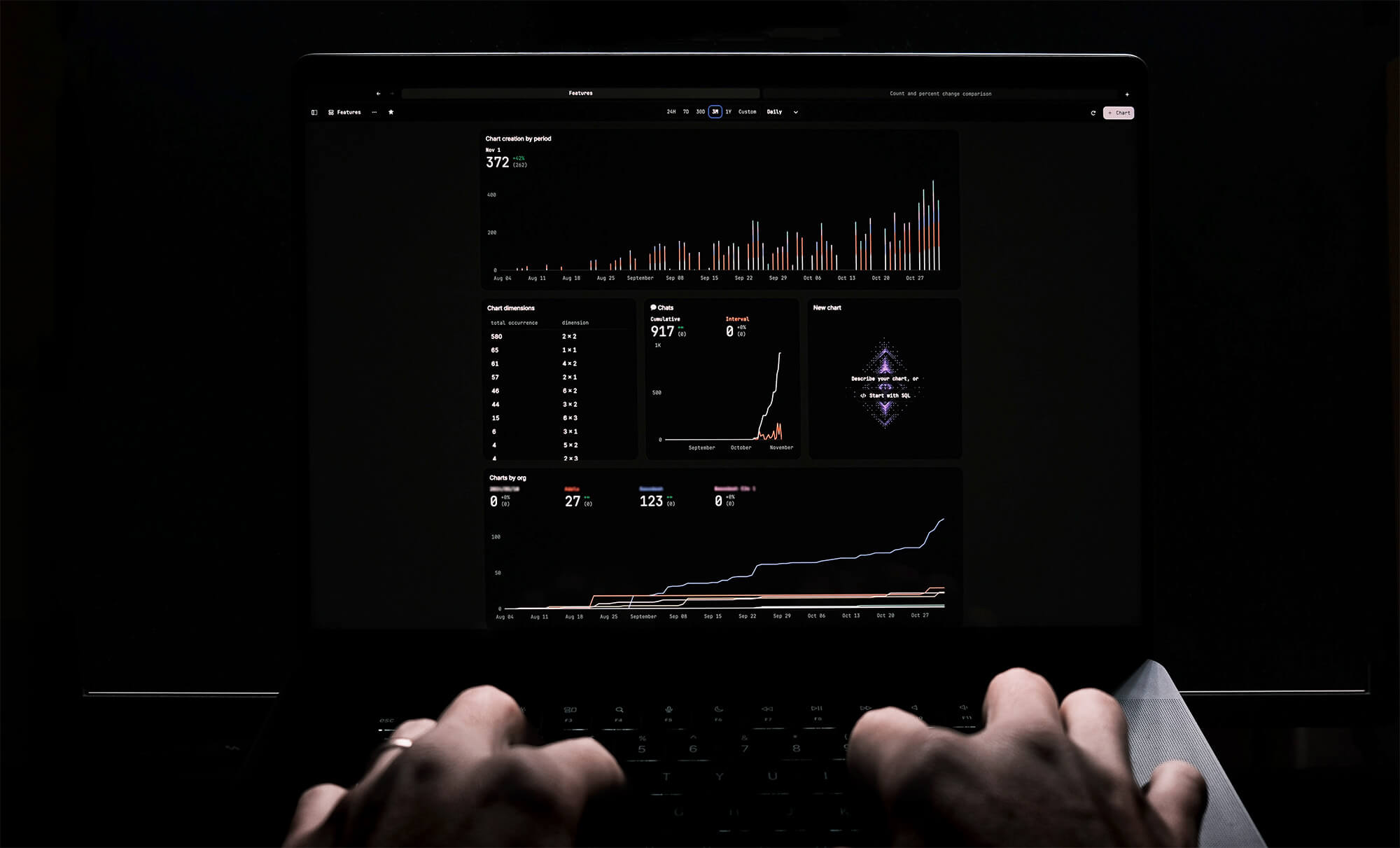
How to Center a Table in HTML with CSS
Jeremy Sarchet
Adjusting HTML Table Column Width for Better Design
Robert Cooper
How to Link Multiple CSS Stylesheets in HTML
Robert Cooper
Mastering HTML Table Inline Styling: A Guide
Max Musing
HTML Multiple Style Attributes: A Quick Guide
Max Musing
How to Set HTML Table Width for Responsive Design
Max Musing