
How to Subtract Dates in TypeScript
When working with TypeScript (or even plain JavaScript), you may occasionally need to subtract two dates to find the difference between them. This guide will help you achieve that, whether you need the difference in milliseconds, seconds, minutes, hours, or days.
1. Basic Date Subtraction in TypeScript
Dates in TypeScript and JavaScript are represented by the Date
object. When you subtract two date objects, you get the difference in milliseconds.
const date1 = new Date("2023-01-01"); const date2 = new Date("2023-01-10"); const differenceInMilliseconds = date2.getTime() - date1.getTime(); console.log(differenceInMilliseconds); // 777600000 (This is 9 days in milliseconds)
2. Getting the Difference in Various Units
Days
To get the difference in days:
const differenceInDays = Math.floor(differenceInMilliseconds / (1000 * 60 * 60 * 24)); console.log(differenceInDays); // 9
Hours
To get the difference in hours:
const differenceInHours = Math.floor(differenceInMilliseconds / (1000 * 60 * 60)); console.log(differenceInHours); // 216 (This is 9 days * 24 hours/day)
Minutes
To get the difference in minutes:
const differenceInMinutes = Math.floor(differenceInMilliseconds / (1000 * 60)); console.log(differenceInMinutes); // 12960 (This is 9 days * 24 hours/day * 60 minutes/hour)
Seconds
To get the difference in seconds:
const differenceInSeconds = Math.floor(differenceInMilliseconds / 1000); console.log(differenceInSeconds); // 777600 (This is 9 days * 24 hours/day * 60 minutes/hour * 60 seconds/minute)
3. Using Third-Party Libraries
Sometimes, working directly with the native Date
object can be cumbersome. For more complex operations or easier date manipulation, you might consider using third-party libraries like moment
or date-fns
.
Using moment
import * as moment from 'moment'; const date1 = moment("2023-01-01"); const date2 = moment("2023-01-10"); const differenceInDays = date2.diff(date1, 'days'); console.log(differenceInDays); // 9
Using date-fns
import { differenceInDays } from 'date-fns'; const date1 = new Date("2023-01-01"); const date2 = new Date("2023-01-10"); const daysDifference = differenceInDays(date2, date1); console.log(daysDifference); // 9
4. Handling Time Zones
Keep in mind that the Date
object in TypeScript/JavaScript uses the system's time zone. If you're working with dates across different time zones, you'll want to ensure consistent and correct calculations. For this, libraries like moment-timezone
or specialized date functions in date-fns
can be beneficial.
In conclusion, subtracting dates in TypeScript can be achieved using the native Date
object or with the help of third-party libraries for more straightforward operations. Remember to handle time zones if necessary.
Invite only
We're building the next generation of data visualization.
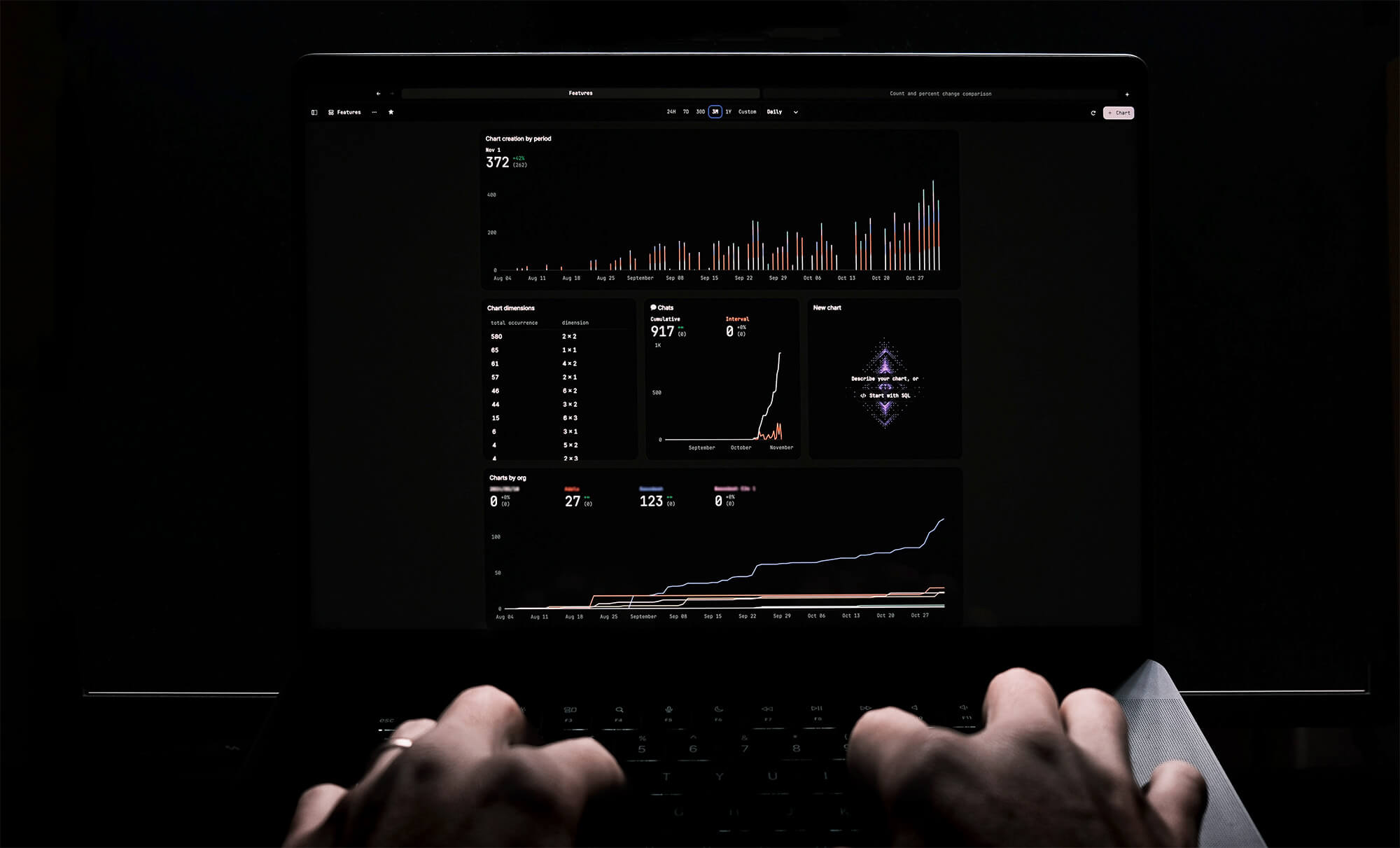
How to turn webpages into editable canvases with a JavaScript bookmarklet
Kris Lachance
How to fix the "not all code paths return a value" issue in TypeScript
Kris Lachance
Working with WebSockets in Node.js using TypeScript
Kris Lachance
Type Annotations Can Only Be Used in TypeScript Files
Kris Lachance
Guide to TypeScript Recursive Type
Kris Lachance
How to Configure Knex.js with TypeScript
Kris Lachance